Unpacking the Either Monad for Elegant Error Handling
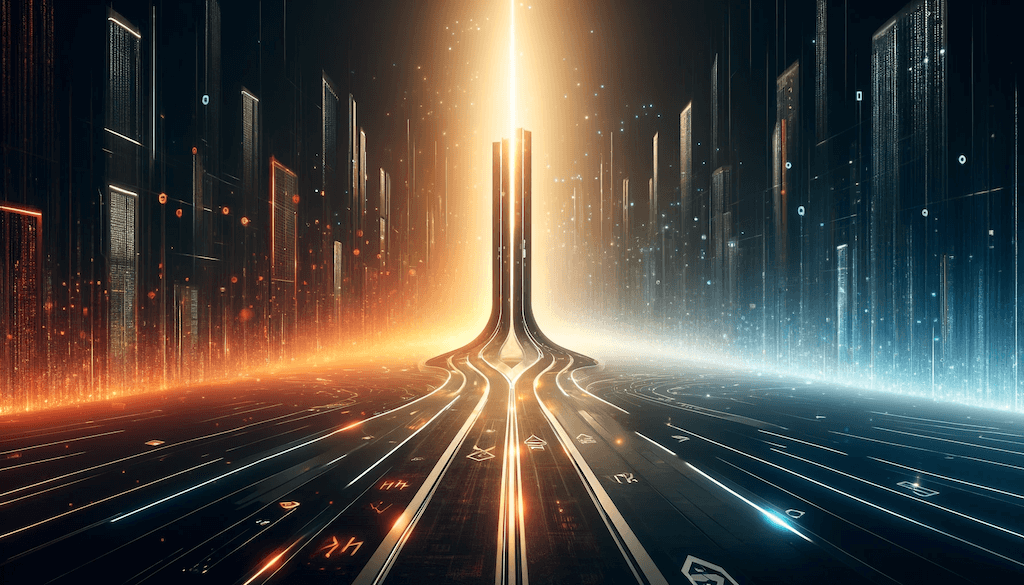
Welcome back to Monadist Monday! In our previous discussions, we explored the Maybe Monad and how it can help manage optional values in your code. This week, we dive into another powerful Monad - the Either Monad. The Either Monad is a versatile tool for handling errors in a clean and efficient way, making it a favorite among functional programmers.
What is the Either Monad?
At its core, the Either Monad represents computations that can result in one of two possible outcomes: a success or a failure. It’s similar to the Maybe Monad, but with an important difference - it provides more information about what went wrong when an error occurs.
The Either Monad is typically used to handle errors in a way that separates successful computations from failures, providing a mechanism to propagate and manage errors without resorting to exceptions.
Structure of the Either Monad
The Either Monad is defined as follows:
Left
: Represents a failure and typically holds an error message or code.Right
: Represents a success and holds the resulting value.
This dual-nature structure allows you to explicitly handle both success and failure cases in your code.
Either Monad in Haskell
Let’s see how the Either Monad is defined and used in Haskell.
data Either a b = Left a | Right b
Here, a
represents the error type, and b
represents the success type.
Using the Either Monad
Consider a scenario where you want to parse an integer from a string. If the string is not a valid integer, you want to return an error message. Here’s how you can do it using the Either Monad.
parseInt :: String -> Either String Int
parseInt str = case reads str of
[(val, "")] -> Right val
_ -> Left "Not a valid integer"
In this example, parseInt
returns either a Right
containing the parsed integer or a Left
containing an error message.
Chaining Computations with the Either Monad
One of the key benefits of using Monads is the ability to chain computations. The Either Monad allows you to chain together multiple operations that may fail, propagating errors as they occur.
Let’s extend our example to include a function that divides two integers, handling the case where the divisor is zero.
divide :: Int -> Int -> Either String Int
divide _ 0 = Left "Division by zero"
divide x y = Right (x `div` y)
safeDivide :: String -> String -> Either String Int
safeDivide strX strY = do
x <- parseInt strX
y <- parseInt strY
divide x y
In the safeDivide
function, we use the do
notation to chain together the parsing and division operations. If any step fails, the error is propagated, and the subsequent steps are skipped.
Practical Example: Handling File Operations
Let’s look at a more practical example where the Either Monad can be used to handle file operations. We’ll write a function to read the contents of a file and return either the contents or an error message if the file does not exist.
import System.IO
import Control.Exception
readFileEither :: FilePath -> IO (Either String String)
readFileEither path = do
result <- try (readFile path) :: IO (Either IOException String)
return $ case result of
Left _ -> Left "File not found"
Right contents -> Right contents
Here, we use the try
function from Control.Exception
to catch any IOException
that might occur during the file read operation. If an exception is caught, we return a Left
with an error message; otherwise, we return a Right
with the file contents.
Advantages of Using the Either Monad
- Explicit Error Handling: The Either Monad makes error handling explicit, improving code readability and maintainability.
- No Exceptions: By using Either, you avoid the pitfalls of exceptions, such as uncaught exceptions and the need for extensive try-catch blocks.
- Composability: The Either Monad allows you to compose multiple computations that may fail, making your code more modular and reusable.
Conclusion
The Either Monad is a powerful tool for handling errors in a clean and expressive way. By using the Either Monad, you can write more robust and maintainable code that clearly separates successful computations from failures.
As you continue your journey into functional programming, mastering the Either Monad will equip you with the skills to handle errors gracefully and effectively.
Stay tuned to hersoncruz.com for more insights and updates on functional programming and other exciting topics. Join us next Monday as we explore another fascinating Monad!
Happy coding!