Supercharge Your Website with Lazy Loading: A Step-by-Step Guide
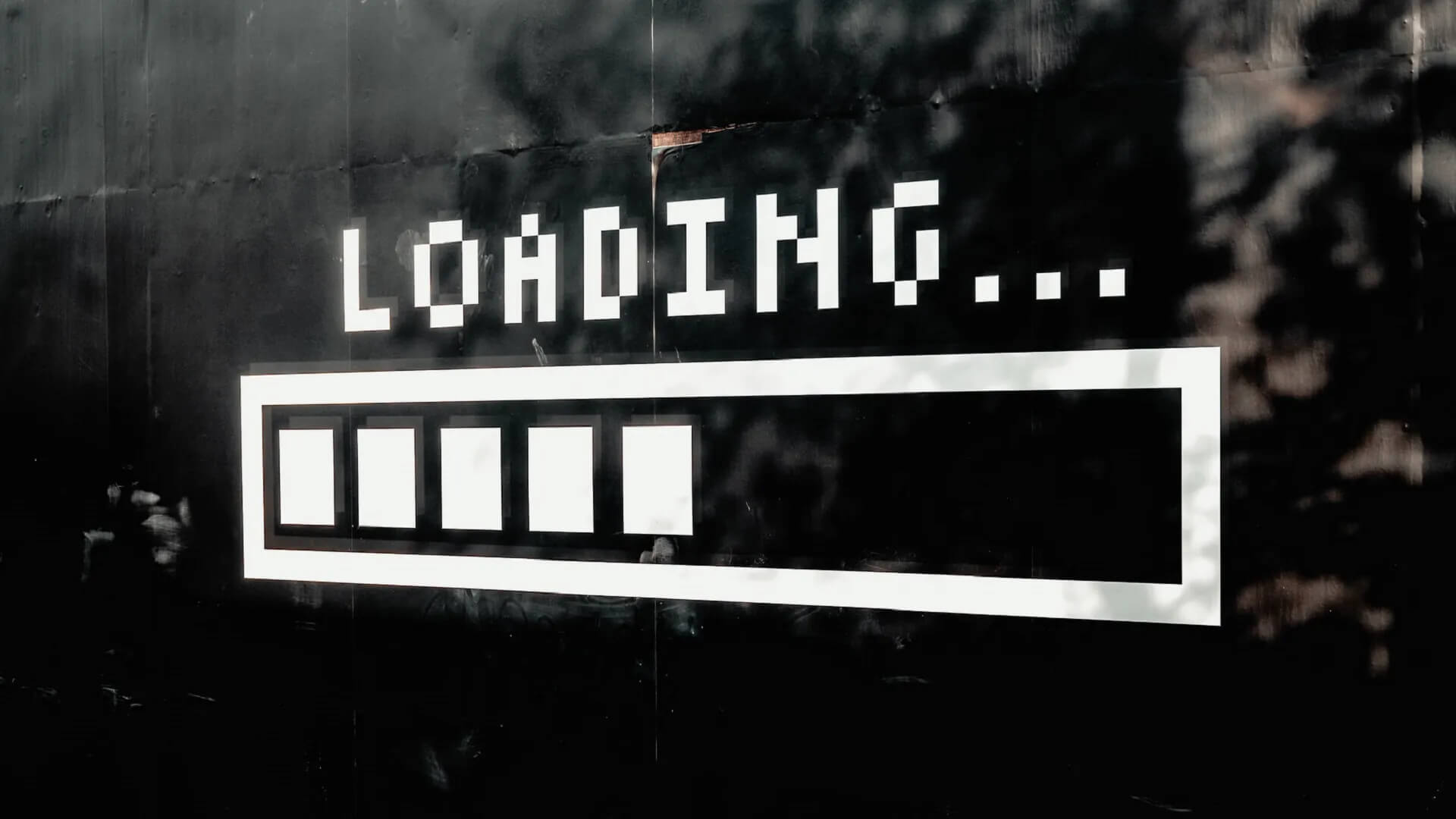
In today’s fast-paced digital world, website speed is more crucial than ever. Not only does a faster site lead to better user engagement, but it also plays a significant role in SEO. One technique that can dramatically enhance your website’s performance is lazy loading.
Lazy loading defers the loading of non-essential resources, such as images and videos, until they are needed. This reduces the initial load time of your web pages, leading to faster performance and improved SEO. In this blog post, we’ll walk you through why lazy loading is important, how it can benefit your website, and the step-by-step process to implement it.
Why Lazy Loading Matters
Lazy loading isn’t just a buzzword—it’s a vital component of modern web development that directly impacts your site’s success. Here’s why:
-
Faster Loading Times: By loading only what’s needed when it’s needed, your website can deliver content faster. This reduces bounce rates and keeps users engaged.
-
Improved SEO: Search engines like Google factor page speed into their ranking algorithms. A faster site can help you rank higher in search results.
-
Enhanced User Experience: Visitors don’t have to wait for unnecessary content to load, making their experience smoother and more enjoyable.
-
Reduced Bandwidth Usage: Lazy loading conserves bandwidth, particularly important for mobile users or those with limited data plans.
Step-by-Step Guide to Implementing Lazy Loading
Step 1: Analyze Your Current Site Performance
Before you start, assess your current website performance. Use tools like Google PageSpeed Insights or GTmetrix to understand how your site performs and identify areas where lazy loading could help.
# Example: Checking site performance with Google PageSpeed Insights
https://developers.google.com/speed/pagespeed/insights/
Step 2: Choose the Right Lazy Loading Method
There are several ways to implement lazy loading, depending on your site’s setup:
- Native Lazy Loading: Supported by modern browsers, this method requires minimal coding.
- JavaScript Libraries: Libraries like
lazysizes
orLozad.js
offer more control and compatibility across different browsers.
For this guide, we’ll use the native method, as it’s straightforward and effective.
Step 3: Implement Native Lazy Loading for Images
HTML’s loading
attribute makes it easy to lazy load images. Simply add loading="lazy"
to your image tags.
<img src="example.jpg" alt="Example Image" loading="lazy">
Step 4: Implement Lazy Loading for Background Images
Lazy loading background images can be trickier, as they are often implemented via CSS. Here’s a JavaScript snippet that helps:
document.addEventListener("DOMContentLoaded", function() {
const lazyBackgrounds = document.querySelectorAll(".lazy-bg");
lazyBackgrounds.forEach(function(bg) {
const observer = new IntersectionObserver(function(entries) {
entries.forEach(entry => {
if (entry.isIntersecting) {
entry.target.style.backgroundImage = `url(${entry.target.dataset.bg})`;
observer.unobserve(entry.target);
}
});
});
observer.observe(bg);
});
});
Use it like this in your HTML:
<div class="lazy-bg" data-bg="background.jpg"></div>
Step 5: Lazy Loading for Videos
For videos, you can lazy load by setting up the src
attribute only when the video is about to be played:
<video controls preload="none" poster="video-poster.jpg">
<source data-src="video.mp4" type="video/mp4">
</video>
<script>
document.addEventListener("DOMContentLoaded", function() {
const videos = document.querySelectorAll("video");
videos.forEach(video => {
video.addEventListener("play", function() {
const source = this.querySelector("source");
if (source.dataset.src) {
source.src = source.dataset.src;
video.load();
}
});
});
});
</script>
Step 6: Test Your Implementation
Once you’ve implemented lazy loading, it’s crucial to test your site to ensure everything works as expected. Use the same performance tools from Step 1 to see the improvements.
Step 7: Monitor and Maintain
Finally, make lazy loading a part of your ongoing site maintenance. Regularly check performance metrics and tweak your implementation as needed.
Conclusion
Lazy loading is a powerful, yet simple technique that can significantly improve your website’s performance and SEO. By following this guide, you’ve taken a crucial step towards creating a faster, more user-friendly website that search engines will love. Implement lazy loading today and watch your site’s traffic and engagement soar!
Ready to Transform Your Website?
For more tips and tricks on web performance and SEO, subscribe to hersoncruz.com and stay ahead of the digital curve.