Unlocking Real-World Superpowers with Monads: Beyond the Code
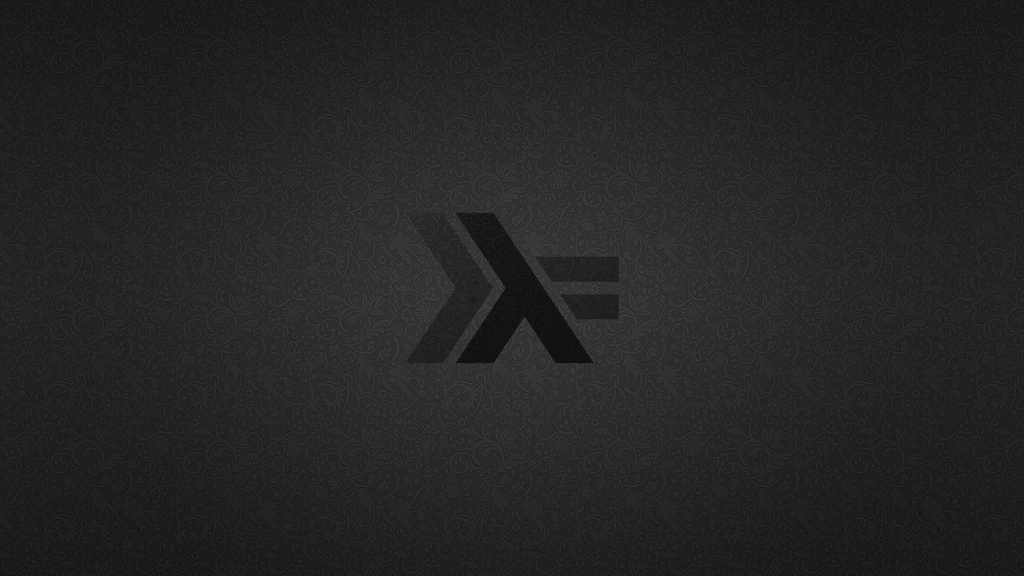
If you’re a seasoned developer, you’ve probably heard of monads. Maybe you’ve even used them in your functional programming adventures. But have you ever considered that these seemingly abstract constructs could be more than just a tool for managing side effects in code? Today, we’re diving deep into the world of monads to uncover how they can be leveraged to solve some of the most complex and fascinating problems in the real world.
What Exactly Are Monads? A Quick Recap
Before we delve into the real-world implications, let’s quickly recap what a monad is. In functional programming, a monad is a design pattern used to handle computations in a sequential manner. Think of it as a “container” that holds a value and allows you to apply functions to that value in a controlled way.
In Haskell, for example, a monad is defined by three core components:
- Type Constructor: This defines the type of the monad, such as
Maybe
,List
, orIO
. - Return Function: This wraps a value into the monad.
- Bind Function: This chains operations, allowing for sequential execution while maintaining the monadic context.
With this quick refresher out of the way, let’s move on to the exciting part: how monads transcend the boundaries of programming to offer solutions in real-world scenarios.
The Power of Monads in Real-World Problem Solving
Monads might seem like abstract mathematical concepts, but they can be powerful tools when applied to real-world problems. Here are some areas where monads are already making a difference, or have the potential to revolutionize problem-solving:
1. Finance: Managing Uncertainty with Maybe Monad
In the world of finance, uncertainty is a constant companion. Whether it’s predicting market trends or evaluating risk, financial models often deal with incomplete or missing data. Enter the Maybe
monad.
The Maybe
monad allows developers to elegantly handle operations that may fail or produce no result, without the need for complex error-handling logic. For instance, in a financial application, calculating the return on investment (ROI) might fail if there’s missing data for some assets. By using the Maybe
monad, the application can continue processing valid data while safely handling missing values, thereby ensuring that the entire computation doesn’t collapse due to a few missing pieces of information.
Example in Haskell:
safeDivide :: Double -> Double -> Maybe Double
safeDivide _ 0 = Nothing
safeDivide x y = Just (x / y)
calculateROI :: [Double] -> Maybe Double
calculateROI [initial, final] = safeDivide (final - initial) initial
calculateROI _ = Nothing
This simple example demonstrates how the Maybe
monad helps in managing uncertainty in financial computations.
2. Cybersecurity: Ensuring Data Integrity with the Writer Monad
Cybersecurity is all about ensuring the integrity and confidentiality of data. The Writer
monad, which allows logging of operations, can be an invaluable tool in this domain. By capturing a log of all actions taken on sensitive data, security systems can maintain a verifiable trail of operations, making it easier to detect anomalies or unauthorized changes.
For example, a system that encrypts and decrypts data could use the Writer
monad to log every encryption and decryption operation, along with metadata about the operation such as timestamps and user IDs. This log can then be analyzed to detect patterns of misuse or attempted breaches.
Example in Haskell:
import Control.Monad.Writer
logEncryption :: String -> Writer [String] String
logEncryption plainText = do
tell ["Encrypting: " ++ plainText]
let encrypted = reverse plainText -- Simple reversal for illustration
tell ["Encrypted: " ++ encrypted]
return encrypted
runLog = runWriter (logEncryption "SensitiveData")
In this case, Writer
helps maintain a detailed log that can be audited for security purposes.
3. Quantum Computing: Managing State with the State Monad
Quantum computing is an emerging field with the potential to revolutionize technology. One of the key challenges in quantum computing is managing the state of qubits—quantum bits that exist in multiple states simultaneously.
The State
monad, which threads state through computations, can be used to model quantum states as they evolve during computation. This approach allows quantum algorithms to be implemented in a functional programming style, making it easier to reason about the complex state transitions that occur during quantum computation.
Example in Haskell:
import Control.Monad.State
type Qubit = (Bool, Bool) -- Simplified for illustration
quantumOperation :: State Qubit Bool
quantumOperation = do
(q1, q2) <- get
let result = q1 && q2 -- Simplified quantum operation
put (result, not result)
return result
runQuantum = runState quantumOperation (True, False)
This example shows how the State
monad can manage the state of qubits in a quantum algorithm, helping bridge the gap between abstract quantum operations and their implementation.
Beyond Code: The Philosophical Implications of Monads
Monads are not just a tool for developers; they embody a philosophy that can be applied to various aspects of life and problem-solving. The idea of chaining operations in a controlled, predictable manner while managing side effects is something that can be extended beyond code.
Consider decision-making processes in business or personal life. The same principles that guide monadic operations—such as managing uncertainty (Maybe
), keeping track of actions (Writer
), or maintaining state (State
)—can also guide how we approach complex decisions.
The Future of Monads in Real-World Applications
As technology evolves, so too will the applications of monads. From AI-driven decision-making systems to complex simulations in physics and biology, the principles of monadic programming will continue to offer elegant solutions to some of the most challenging problems.
The future may see the development of new monads specifically designed for emerging technologies, such as the Blockchain
monad for handling decentralized transactions, or the NeuralNet
monad for managing the state of machine learning models. The possibilities are as vast as the problems we face, and monads will likely play a key role in the solutions of tomorrow.
Conclusion: Embracing the Power of Monads Beyond Programming
Monads are often seen as a challenging concept to grasp, but once understood, they open up a world of possibilities beyond the realm of code. Whether it’s managing uncertainty in finance, ensuring data integrity in cybersecurity, or modeling quantum states, monads provide a powerful framework for solving complex problems.
As we continue to explore the potential of monads, both in code and in real life, we unlock new ways to approach challenges with clarity, precision, and elegance. The next time you encounter a tough problem—whether it’s in software development or beyond—consider how monads might help you break it down, manage the complexities, and find a solution.
So, are you ready to embrace the monadic mindset and unlock your real-world superpowers?