Streamlining File Management with Python
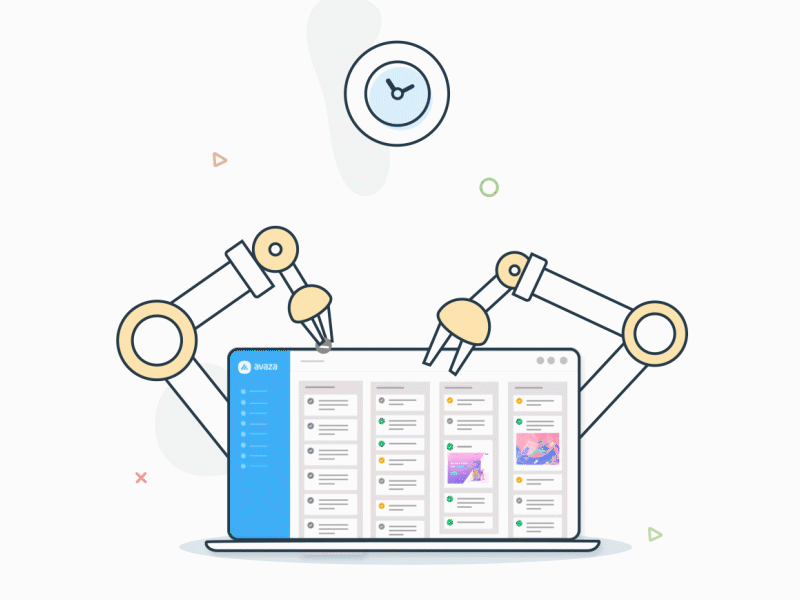
Welcome to another installment of Task Automation Tuesday! Today, we are going to explore how you can automate file management tasks using Python. Whether you are a seasoned sysadmin or just starting out, these scripts will help you save time and increase your productivity by automating repetitive file management tasks.
Why Automate File Management?
Managing files manually can be time-consuming and prone to errors, especially when dealing with large volumes of data. Automation can help you:
- Organize files more efficiently
- Backup important data regularly
- Ensure consistency and reduce the risk of human error
Let’s dive into some practical Python scripts that can help you streamline your file management tasks.
Script 1: Organizing Files by Extension
One common task is organizing files into folders based on their extensions. This script will scan a directory and move files into subdirectories according to their file types.
import os
import shutil
def organize_files_by_extension(directory):
for filename in os.listdir(directory):
if os.path.isfile(os.path.join(directory, filename)):
extension = filename.split('.')[-1]
folder_path = os.path.join(directory, extension)
if not os.path.exists(folder_path):
os.makedirs(folder_path)
shutil.move(os.path.join(directory, filename), folder_path)
if __name__ == "__main__":
organize_files_by_extension('/path/to/your/directory')
Script 2: Automated Backups
Regular backups are essential to prevent data loss. This script creates a backup of a specified directory and saves it with a timestamp.
import os
import shutil
import datetime
def backup_directory(source_directory, backup_directory):
timestamp = datetime.datetime.now().strftime("%Y%m%d%H%M%S")
backup_path = os.path.join(backup_directory, f"backup_{timestamp}")
shutil.copytree(source_directory, backup_path)
print(f"Backup created at {backup_path}")
if __name__ == "__main__":
backup_directory('/path/to/source_directory', '/path/to/backup_directory')
Script 3: Cleaning Up Old Files
Over time, directories can accumulate a lot of old files that are no longer needed. This script deletes files older than a specified number of days.
import os
import time
def delete_old_files(directory, days):
now = time.time()
cutoff = now - (days * 86400)
for filename in os.listdir(directory):
file_path = os.path.join(directory, filename)
if os.path.isfile(file_path) and os.path.getmtime(file_path) < cutoff:
os.remove(file_path)
print(f"Deleted {file_path}")
if __name__ == "__main__":
delete_old_files('/path/to/your/directory', 30)
Conclusion
Automating file management tasks with Python can significantly enhance your productivity and ensure that your files are well-organized and safe. These scripts are just the beginning—there are countless ways you can leverage Python to automate your daily tasks. Experiment with these examples, customize them to fit your needs, and enjoy the benefits of a more streamlined workflow.
Stay tuned for more automation tips and tricks next Tuesday. Happy scripting!