Monadist Monday: Understanding the Maybe Monad
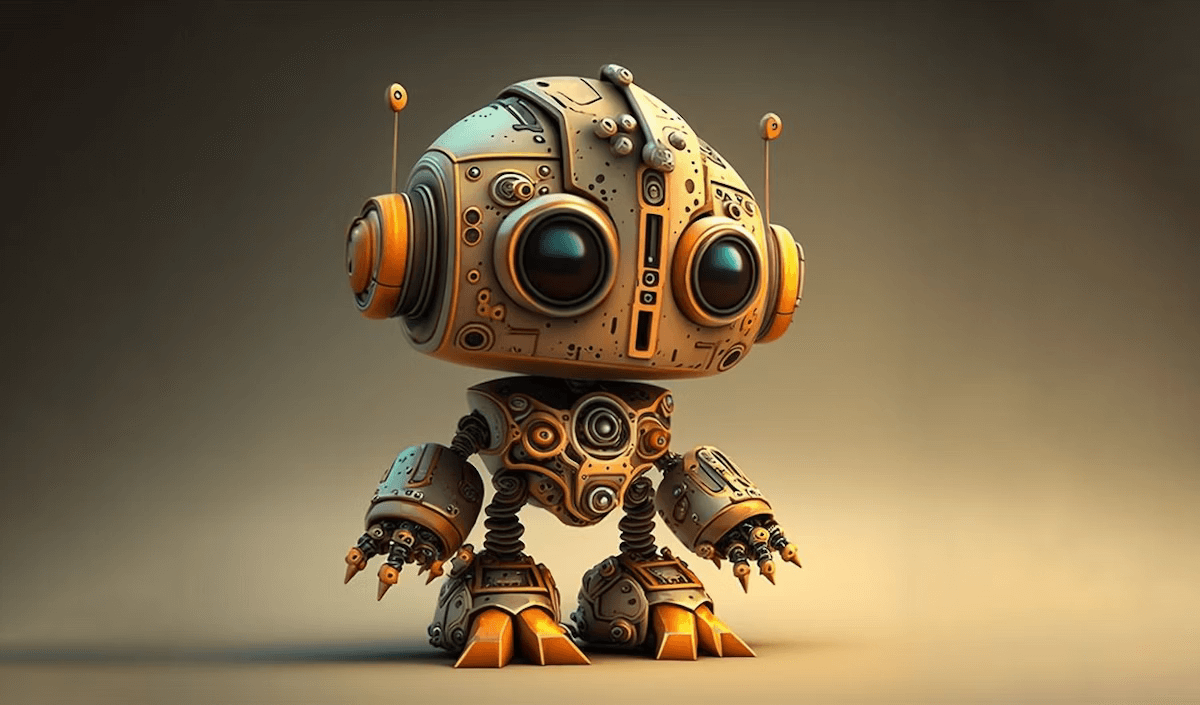
Welcome back to Monadist Monday! In our second post of this series, we will take a closer look at the Maybe monad. We introduced the concept of monads last week, and today we will explore how the Maybe monad can help handle optional values and errors in a clean and functional way. Let’s dive in!
What is the Maybe Monad?
The Maybe monad is a common pattern in functional programming that deals with computations that might fail or return nothing. It helps us avoid null reference errors and provides a more elegant way to handle optional values.
In essence, the Maybe monad can be in one of two states:
- Just: Represents a value.
- Nothing: Represents the absence of a value.
Why Use the Maybe Monad?
Using the Maybe monad allows us to write safer code by explicitly handling cases where a value might be absent. This reduces the likelihood of encountering null reference errors and makes our code more predictable and easier to reason about.
Benefits of the Maybe Monad
- Explicit Handling: Forces us to explicitly handle the absence of a value.
- Chaining Operations: Allows us to chain operations without checking for null values at each step.
- Cleaner Code: Reduces the need for nested if-else statements and null checks.
Implementing the Maybe Monad in Python
Let’s start by implementing a simple version of the Maybe monad in Python.
class Maybe:
def __init__(self, value):
self.value = value
def is_nothing(self):
return self.value is None
def bind(self, func):
if self.is_nothing():
return self
return func(self.value)
def just(value):
return Maybe(value)
def nothing():
return Maybe(None)
Using the Maybe Monad
Now that we have our Maybe monad implemented, let’s see how we can use it to handle optional values.
def get_value(d, key):
return just(d.get(key)) if key in d else nothing()
data = {'a': 1, 'b': 2}
result = get_value(data, 'a').bind(lambda x: just(x + 1))
print(result.value) # Output: 2
result = get_value(data, 'c').bind(lambda x: just(x + 1))
print(result.value) # Output: None
In this example, we use the get_value
function to retrieve a value from a dictionary. If the key exists, we wrap the value in a Just
monad; otherwise, we return Nothing
. We then use the bind
method to chain operations on the value, ensuring that we handle the absence of the value gracefully.
Enhancing the Maybe Monad
Let’s add some additional methods to our Maybe monad to make it more powerful and easier to use.
class Maybe:
def __init__(self, value):
self.value = value
def is_nothing(self):
return self.value is None
def bind(self, func):
if self.is_nothing():
return self
return func(self.value)
def map(self, func):
if self.is_nothing():
return self
return just(func(self.value))
def get_or_else(self, default):
if self.is_nothing():
return default
return self.value
def just(value):
return Maybe(value)
def nothing():
return Maybe(None)
Now, let’s use these new methods in a practical example.
def safe_divide(a, b):
return just(a / b) if b != 0 else nothing()
result = safe_divide(10, 2).map(lambda x: x * 2).get_or_else("Cannot divide by zero")
print(result) # Output: 10.0
result = safe_divide(10, 0).map(lambda x: x * 2).get_or_else("Cannot divide by zero")
print(result) # Output: Cannot divide by zero
In this example, we define a safe_divide
function that returns a Just
monad if the division is possible and Nothing
if the divisor is zero. We then use the map
method to apply a function to the result and the get_or_else
method to provide a default value if the result is Nothing
.
Conclusion
The Maybe monad is a powerful tool for handling optional values and errors in a functional programming style. By using the Maybe monad, we can write cleaner, safer, and more maintainable code. In this post, we explored how to implement and use the Maybe monad in Python, and we saw how it can help us handle optional values gracefully.
Stay tuned for next week’s Monadist Monday post, where we will dive into another exciting monad and explore more advanced functional programming concepts. Happy coding!