Monadist Monday: Diving Deeper into the Maybe Monad
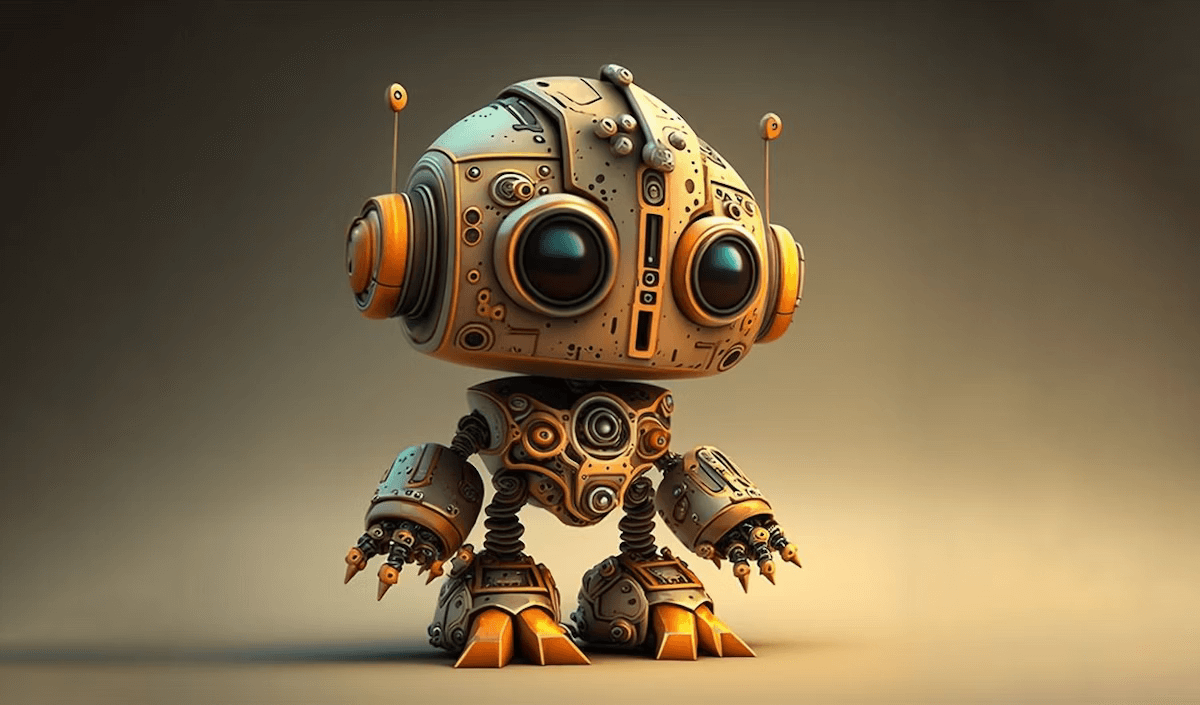
Welcome back to Monadist Monday! In our previous post, we introduced the concept of the Maybe Monad in Python, a powerful tool for handling computations that might fail or return nothing. Today, we’re going to dive deeper into advanced uses of the Maybe Monad and see how it can simplify error handling and manage optional values in your code. Grab your coffee and let’s get started!
Recap: The Maybe Monad
As a quick refresher, the Maybe Monad represents computations that might fail. It has two possible values: Just
(which holds a value) and Nothing
(which represents the absence of a value). This allows us to chain computations together without having to check for None
at every step.
Here’s the basic implementation we covered last time:
class Maybe:
def __init__(self, value=None):
self.value = value
def is_nothing(self):
return self.value is None
def bind(self, func):
if self.is_nothing():
return self
try:
return func(self.value)
except Exception as e:
return Nothing()
def __repr__(self):
if self.is_nothing():
return "Nothing"
else:
return f"Just({self.value})"
def Just(value):
return Maybe(value)
def Nothing():
return Maybe()
Advanced Use Case: Nested Maybe Monads
One of the common scenarios where the Maybe Monad shines is in handling nested optionals. Consider a function that fetches a user from a database, which might return None
, and another function that fetches the user’s profile, which might also return None
.
Here’s how we can handle this scenario using the Maybe Monad:
def fetch_user(user_id):
# Simulate fetching a user from the database
users = {1: 'Alice', 2: 'Bob'}
return just(users.get(user_id)) if user_id in users else nothing()
def fetch_profile(user):
# Simulate fetching a user's profile
profiles = {'Alice': 'Profile of Alice', 'Bob': 'Profile of Bob'}
return just(profiles.get(user)) if user in profiles else nothing()
# Chaining the operations
user_id = 1
profile = fetch_user(user_id).bind(fetch_profile)
print(profile.value) # Output: Profile of Alice
Simplifying Error Handling
Another powerful application of the Maybe Monad is in simplifying error handling. By using the Maybe Monad, we can avoid deeply nested if-else statements and make our code more readable and maintainable.
Consider a series of functions that might fail at any point:
def step1(x):
return just(x + 1) if x < 10 else nothing()
def step2(x):
return just(x * 2) if x % 2 == 0 else nothing()
def step3(x):
return just(x - 5) if x > 0 else nothing()
# Chaining the steps
result = just(3).bind(step1).bind(step2).bind(step3)
print(result.value) # Output: 2
Combining Maybe with Other Monads
Monads can be combined to handle more complex scenarios. Let’s see how we can combine the Maybe Monad with a simple Result Monad that represents a computation that might fail with an error message.
class Result:
def __init__(self, value, is_error=False):
self.value = value
self.is_error = is_error
def bind(self, func):
if self.is_error:
return self
return func(self.value)
def success(value):
return Result(value)
def failure(message):
return Result(message, is_error=True)
def step1(x):
return success(x + 1) if x < 10 else failure("Step 1 failed")
def step2(x):
return success(x * 2) if x % 2 == 0 else failure("Step 2 failed")
def step3(x):
return success(x - 5) if x > 0 else failure("Step 3 failed")
# Combining Result Monad with Maybe Monad
maybe_result = just(3).bind(step1).bind(step2).bind(step3)
if maybe_result.is_nothing():
print("Computation failed")
else:
print(maybe_result.value)
Conclusion
The Maybe Monad is a versatile tool in functional programming that can simplify error handling and manage optional values effectively. By chaining computations together, we can avoid nested if-else statements and write more readable and maintainable code.
Stay tuned for more Monadist Monday posts, where we’ll continue to explore the fascinating world of monads and functional programming. Happy coding!