Automating Security Audits with Bash Scripts
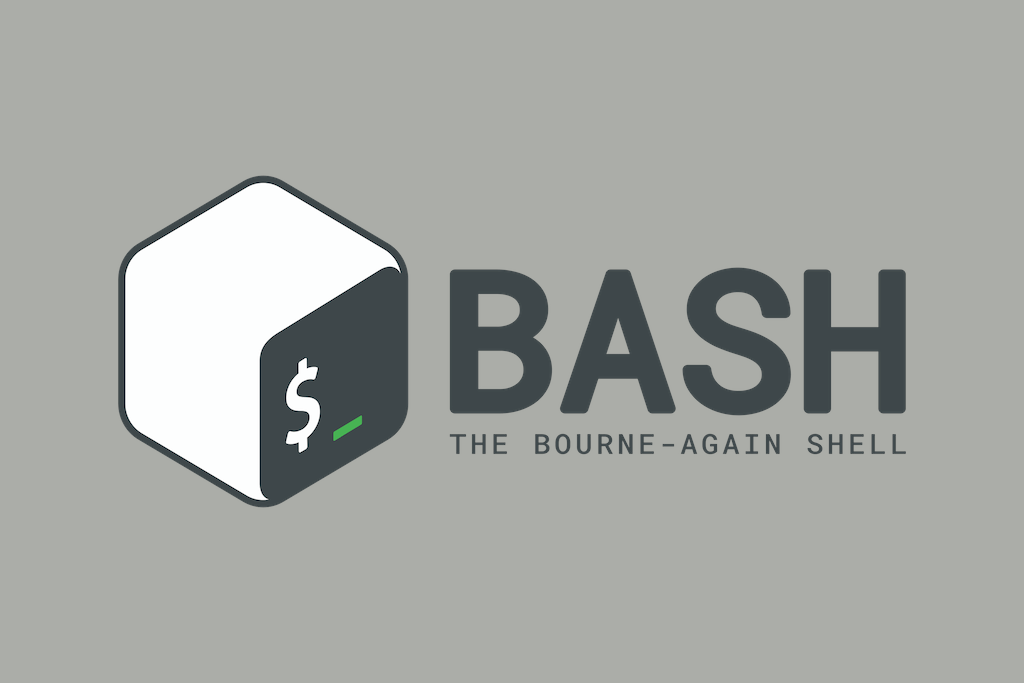
Learn how to automate security audits using Bash scripts to ensure your systems remain secure and compliant. Perfect for both seasoned and novice sysadmins.
Introduction
Welcome to another edition of “Saturday Scripting”! This week, we’re diving into the world of security audits and how you can leverage the power of Bash scripts to automate this critical task. Security audits are essential for maintaining the integrity and compliance of your systems. However, they can be time-consuming and prone to human error. Automating these audits not only saves time but also ensures consistency and thoroughness.
Why Automate Security Audits?
- Consistency: Automated scripts run the same way every time, ensuring that no steps are missed.
- Efficiency: Save hours of manual work by automating repetitive tasks.
- Proactivity: Regular automated audits help identify issues before they become serious problems.
- Documentation: Automated scripts provide a clear record of what checks were performed and when.
Setting Up Your Bash Script
Let’s create a Bash script to perform some common security audit tasks. This script will check for common vulnerabilities and configuration issues, providing a summary of the findings.
Step 1: Checking for Open Ports
Open ports can be an entry point for attackers. We’ll use netstat
to list open ports.
#!/bin/bash
echo "Checking for open ports..."
netstat -tuln
echo "Open ports check complete."
Step 2: Verifying User Accounts
Ensure there are no unauthorized user accounts, empty password fields, root access accounts, or inactive accounts.
echo "Checking for user accounts..."
# List all user accounts
echo "All user accounts:"
cat /etc/passwd | awk -F: '{ print $1 }' > users.txt
cat users.txt
# Check for accounts with empty password fields
echo
echo "Checking for accounts with empty password fields..."
awk -F: '($2 == "") { print $1 }' /etc/shadow > empty_passwords.txt
if [ -s empty_passwords.txt ]; then
echo "Accounts with empty passwords:"
cat empty_passwords.txt
else
echo "No accounts with empty passwords."
fi
# Check for accounts with UID 0 (root access)
echo
echo "Checking for accounts with root access..."
awk -F: '($3 == "0") { print $1 }' /etc/passwd > root_accounts.txt
if [ -s root_accounts.txt ]; then
echo "Accounts with root access:"
cat root_accounts.txt
else
echo "No accounts with root access."
fi
# Check for disabled accounts
echo
echo "Checking for disabled accounts..."
awk -F: '($7 == "/usr/sbin/nologin" || $7 == "/bin/false") { print $1 }' /etc/passwd > disabled_accounts.txt
if [ -s disabled_accounts.txt ]; then
echo "Disabled accounts:"
cat disabled_accounts.txt
else
echo "No disabled accounts."
fi
# Check for last login date
echo
echo "Checking last login date for each user..."
lastlog | grep -v "Never logged in" > last_login.txt
if [ -s last_login.txt ]; then
echo "Last login dates:"
cat last_login.txt
else
echo "No users have logged in."
fi
echo "User accounts check complete."
Step 3: Checking for World-Writable Files
World-writable files can be a security risk. We’ll find and list them.
echo "Checking for world-writable files..."
find / -type f -perm -o+w -exec ls -l {} \; > world_writable_files.txt
echo "World-writable files check complete."
Step 4: Ensuring SELinux or AppArmor is Enabled
SELinux or AppArmor provides an additional layer of security. We’ll check if either is enabled.
echo "Checking for SELinux/AppArmor status..."
if command -v getenforce &> /dev/null
then
selinux_status=$(getenforce)
echo "SELinux is $selinux_status"
else
echo "SELinux is not installed."
fi
if command -v aa-status &> /dev/null
then
apparmor_status=$(aa-status)
echo "AppArmor is enabled: $apparmor_status"
else
echo "AppArmor is not installed."
fi
echo "SELinux/AppArmor check complete."
Step 5: Summarizing the Results
Finally, we’ll summarize the results of our security audit.
echo "Security Audit Summary:"
echo "========================="
echo "Open Ports:"
netstat -tuln
echo
echo "User Accounts:"
cat users.txt
echo
echo "Empty Passwords:"
cat empty_paswords.txt
echo
echo "Root Accounts:"
cat root_accounts.txt
echo
echo "Disabled Accounts:"
cat disabled_accounts.txt
echo
echo "Last Login"
cat last_login.txt
echo
echo "World-Writable Files:"
cat world_writable_files.txt
echo
echo "SELinux/AppArmor Status:"
if command -v getenforce &> /dev/null
then
echo "SELinux is $selinux_status"
else
echo "SELinux is not installed."
fi
if command -v aa-status &> /dev/null
then
echo "AppArmor is enabled: $apparmor_status"
else
echo "AppArmor is not installed."
fi
echo "========================="
echo "Security audit completed successfully."
Running Your Security Audit Script
Save the script as security_audit.sh
and make it executable:
chmod +x security_audit.sh
Run the script with:
./security_audit.sh
The script will perform the security checks and provide a summary of the results. You can schedule this script to run at regular intervals using cron to ensure continuous monitoring.
Conclusion
Automating security audits with Bash scripts is a powerful way to enhance your system’s security posture. By incorporating these scripts into your regular maintenance routines, you can proactively identify and address potential vulnerabilities, ensuring your systems remain secure and compliant. Stay tuned for more scripting tips and tricks next Saturday!
Stay updated with the latest in scripting and sysadmin tips at hersoncruz.com. Happy scripting!