Automate Your Cybersecurity with Python: A Powerful Script to Protect Your Server in Real-Time
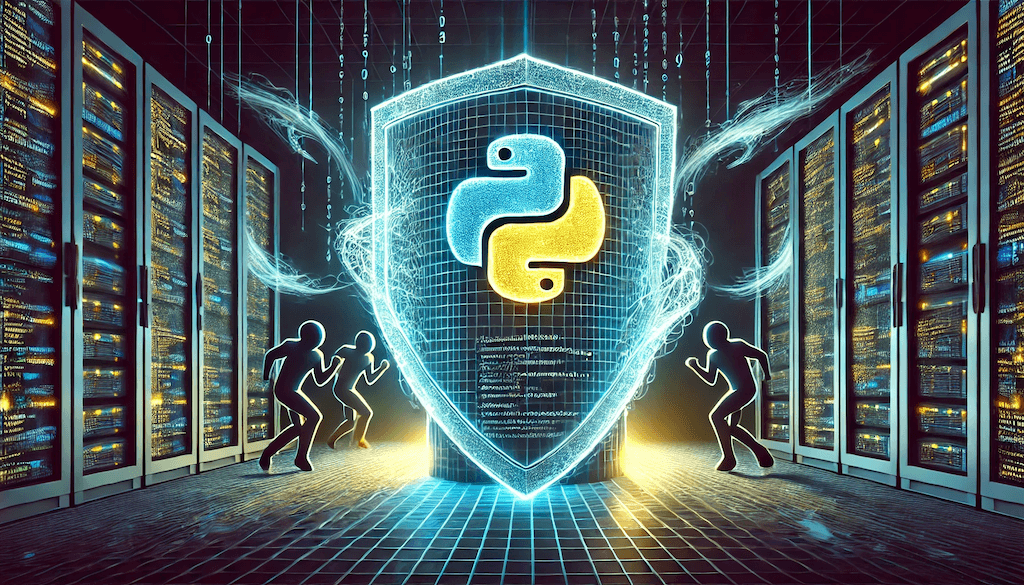
In the rapidly evolving world of cybersecurity, staying ahead of potential threats is crucial. As cyber-attacks become more sophisticated, it’s essential to implement real-time security measures that can adapt and respond automatically. What if you could automate your server’s cybersecurity with a Python script that continuously monitors and reacts to threats? This post will show you how.
Why Automate Cybersecurity?
Cybersecurity automation is not just a trend; it’s a necessity. With the increasing frequency of attacks and the complexity of modern threats, manual monitoring and response are no longer sufficient. Automation allows for:
- Real-Time Monitoring: Continuously watch for suspicious activity without human intervention.
- Instant Response: Automatically block or mitigate threats as soon as they are detected.
- Efficiency: Reduce the workload on your IT team by handling routine security tasks automatically.
- Scalability: Easily scale your security measures across multiple servers.
The Power of Python for Cybersecurity Automation
Python is a versatile language that’s widely used in cybersecurity due to its simplicity and powerful libraries. By leveraging Python, you can create a robust script that monitors your server in real-time, identifies potential threats, and takes immediate action.
Building the Script: Step-by-Step Guide
Let’s dive into the script that will automate your server’s cybersecurity. We’ll use Python along with some key libraries to achieve this.
Step 1: Set Up Your Environment
Before starting, make sure you have Python installed on your server. You can install the required libraries using pip:
pip install requests paramiko python-nmap
Step 2: Monitor Server Logs in Real-Time
We’ll use Python to monitor server logs for any suspicious activity. This includes failed login attempts, unauthorized access, and other anomalies.
import time
import os
LOG_FILE = "/var/log/auth.log" # Path to your server's log file
def tail(f):
f.seek(0, os.SEEK_END)
while True:
line = f.readline()
if not line:
time.sleep(0.1)
continue
yield line
def monitor_logs():
with open(LOG_FILE, 'r') as f:
loglines = tail(f)
for line in loglines:
if "Failed password" in line or "unauthorized" in line.lower():
print(f"Suspicious activity detected: {line}")
take_action(line)
def take_action(log_line):
ip_address = extract_ip(log_line)
if ip_address:
block_ip(ip_address)
def extract_ip(log_line):
# Basic example, adapt as needed
import re
match = re.search(r'[0-9]+(?:\.[0-9]+){3}', log_line)
return match.group(0) if match else None
def block_ip(ip):
os.system(f"iptables -A INPUT -s {ip} -j DROP")
print(f"Blocked IP: {ip}")
if __name__ == "__main__":
monitor_logs()
Step 3: Real-Time Network Scanning
Enhance your security by adding real-time network scanning. This helps detect unauthorized devices or unusual network traffic.
import nmap
def scan_network():
nm = nmap.PortScanner()
nm.scan(hosts='192.168.1.0/24', arguments='-sn')
for host in nm.all_hosts():
if nm[host].state() == "up":
print(f"Host {host} is up")
check_host(host)
def check_host(host):
if host not in trusted_hosts:
print(f"Unknown host detected: {host}")
block_ip(host)
trusted_hosts = ['192.168.1.1', '192.168.1.2'] # Add your trusted IPs here
if __name__ == "__main__":
while True:
scan_network()
time.sleep(300) # Scan every 5 minutes
Step 4: Integrate with Threat Intelligence
By integrating threat intelligence, you can enhance your script’s ability to identify known malicious IPs and domains.
import requests
THREAT_INTEL_API = "https://api.threatintelligenceplatform.com/v1/ip"
API_KEY = "YOUR_API_KEY"
def check_threat_intel(ip):
response = requests.get(f"{THREAT_INTEL_API}/{ip}?apiKey={API_KEY}")
if response.status_code == 200:
data = response.json()
if data['malicious']:
print(f"Malicious IP detected: {ip}")
block_ip(ip)
if __name__ == "__main__":
monitor_logs()
scan_network()
Step 5: Notifications and Alerts
Finally, set up notifications so that you are alerted to any significant threats or actions taken by the script.
import smtplib
from email.mime.text import MIMEText
def send_alert(message):
msg = MIMEText(message)
msg['Subject'] = 'Security Alert'
msg['From'] = 'your_email@example.com'
msg['To'] = 'admin@example.com'
with smtplib.SMTP('smtp.example.com') as server:
server.login('your_email@example.com', 'password')
server.send_message(msg)
print("Alert sent!")
def take_action(log_line):
ip_address = extract_ip(log_line)
if ip_address:
block_ip(ip_address)
send_alert(f"Blocked IP: {ip_address}")
if __name__ == "__main__":
monitor_logs()
Conclusion
With this powerful Python script, you’ve automated the cybersecurity of your server. This script monitors your logs, scans your network, and integrates threat intelligence—all in real-time. It’s an efficient, scalable solution that can save you time and protect your digital assets from potential threats.
As cyber threats continue to evolve, staying ahead with automated solutions like this is essential. Share this script with your network, and let’s collectively raise the bar in cybersecurity!