Automate Suspicious Network Activity Detection with Python
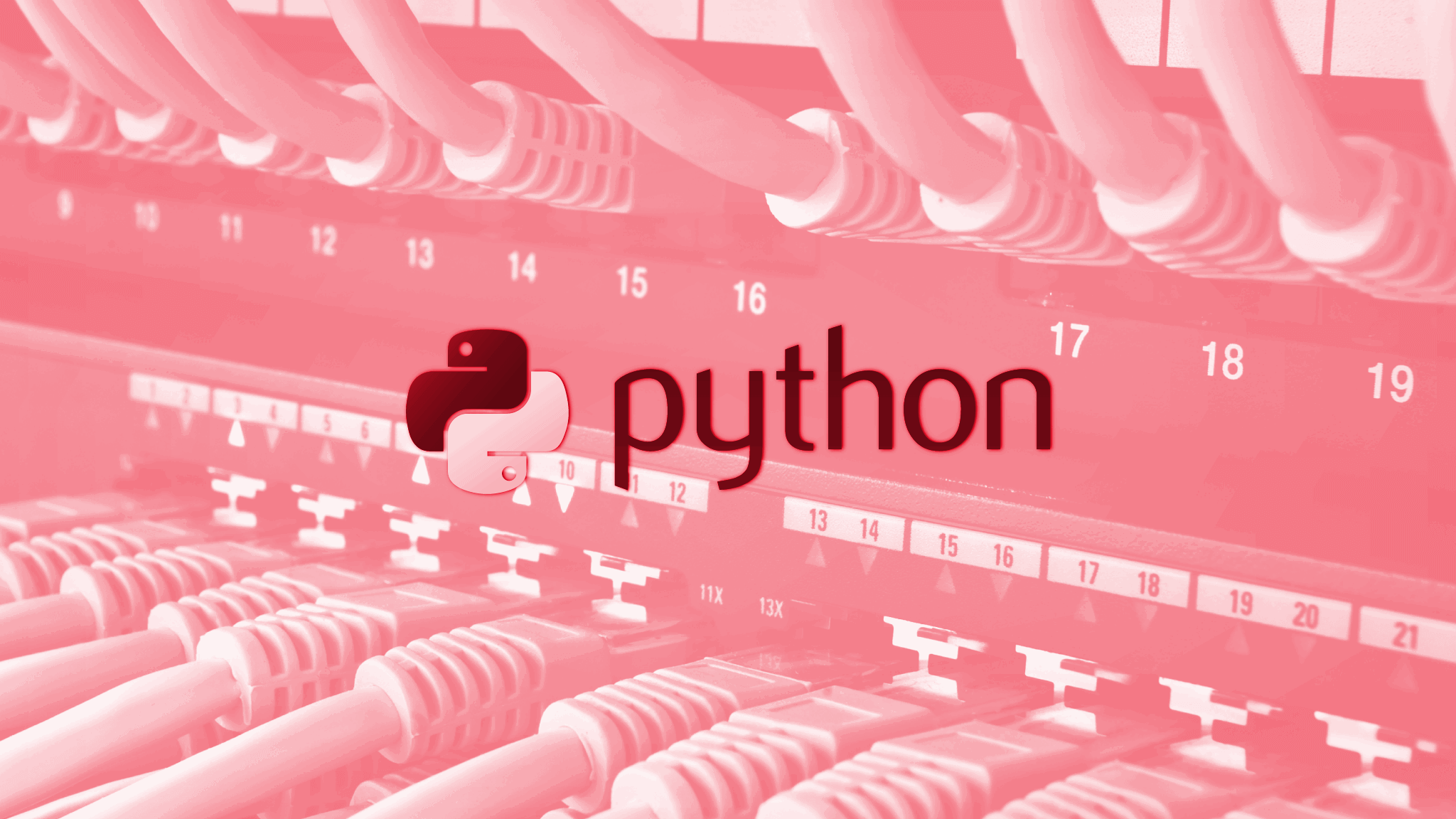
Welcome back to another edition of Saturday Scripting! This week, we’re diving into the world of cybersecurity with a script that will help you automate the detection of suspicious network activity. This is a must-have for any sysadmin looking to bolster their server’s security. So, grab a coffee, and let’s get hacking!
Why Network Monitoring Matters
Network monitoring is crucial for identifying potential threats and unusual activities. By keeping an eye on network traffic, you can detect and respond to security incidents before they escalate. Today, we’ll create a Python script that monitors network traffic and alerts you to any suspicious activity.
The Plan
Our script will:
- Capture network packets.
- Analyze the packets for suspicious patterns.
- Alert you via email if any suspicious activity is detected.
Setting Up the Environment
First, ensure you have Python installed on your system. We’ll also need the scapy
and smtplib
libraries. Install them using pip:
pip install scapy
The Script
Let’s start scripting! Open your favorite text editor and create a new Python file named network_monitor.py
.
#!/usr/bin/env python3
from scapy.all import *
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
# Configuration
ALERT_EMAIL = "your-email@example.com"
SMTP_SERVER = "smtp.example.com"
SMTP_PORT = 587
SMTP_USERNAME = "your-email@example.com"
SMTP_PASSWORD = "your-email-password"
# Suspicious patterns (simple example)
SUSPICIOUS_PATTERNS = [
{"pattern": b"malicious", "description": "Possible malicious traffic"},
{"pattern": b"exploit", "description": "Possible exploit attempt"},
]
def send_alert(subject, body):
msg = MIMEMultipart()
msg["From"] = SMTP_USERNAME
msg["To"] = ALERT_EMAIL
msg["Subject"] = subject
msg.attach(MIMEText(body, "plain"))
try:
server = smtplib.SMTP(SMTP_SERVER, SMTP_PORT)
server.starttls()
server.login(SMTP_USERNAME, SMTP_PASSWORD)
server.sendmail(SMTP_USERNAME, ALERT_EMAIL, msg.as_string())
server.quit()
print(f"Alert sent: {subject}")
except Exception as e:
print(f"Failed to send alert: {e}")
def detect_suspicious(packet):
if packet.haslayer(Raw):
payload = packet[Raw].load
for pattern in SUSPICIOUS_PATTERNS:
if pattern["pattern"] in payload:
description = pattern["description"]
src_ip = packet[IP].src
dst_ip = packet[IP].dst
alert_subject = f"Suspicious Activity Detected: {description}"
alert_body = f"Source IP: {src_ip}\nDestination IP: {dst_ip}\nDescription: {description}"
send_alert(alert_subject, alert_body)
break
def main():
print("Starting network monitor...")
sniff(prn=detect_suspicious, store=0)
if __name__ == "__main__":
main()
How It Works
- Configuration: Replace the placeholder values in the configuration section with your email and SMTP server details.
- Patterns: Define patterns that you consider suspicious in the
SUSPICIOUS_PATTERNS
list. - Packet Sniffing: The
sniff
function fromscapy
captures network packets and calls thedetect_suspicious
function for each packet. - Suspicious Detection: The
detect_suspicious
function checks if any defined pattern is present in the packet’s payload. If found, it sends an alert email.
Running the Script
To run the script, execute:
sudo python3 network_monitor.py
Make sure to run the script with sufficient privileges to capture network traffic.
Enhancements
- Advanced Patterns: Expand the
SUSPICIOUS_PATTERNS
with more complex patterns or integrate with external threat intelligence feeds. - Logging: Implement logging to a file for better auditability and historical analysis.
- Real-time Dashboard: Integrate with tools like Grafana for a real-time monitoring dashboard.
Conclusion
And there you have it! A powerful and hacky Python script to help you monitor network traffic and detect suspicious activities. This tool can be a valuable addition to your cybersecurity arsenal, helping you stay one step ahead of potential threats.
Stay tuned for more exciting and useful scripts in our Saturday Scripting series. Until next time, happy scripting!