Advanced Web Security: Automating CSP, SRI, and Security Headers in Your CI/CD Pipeline
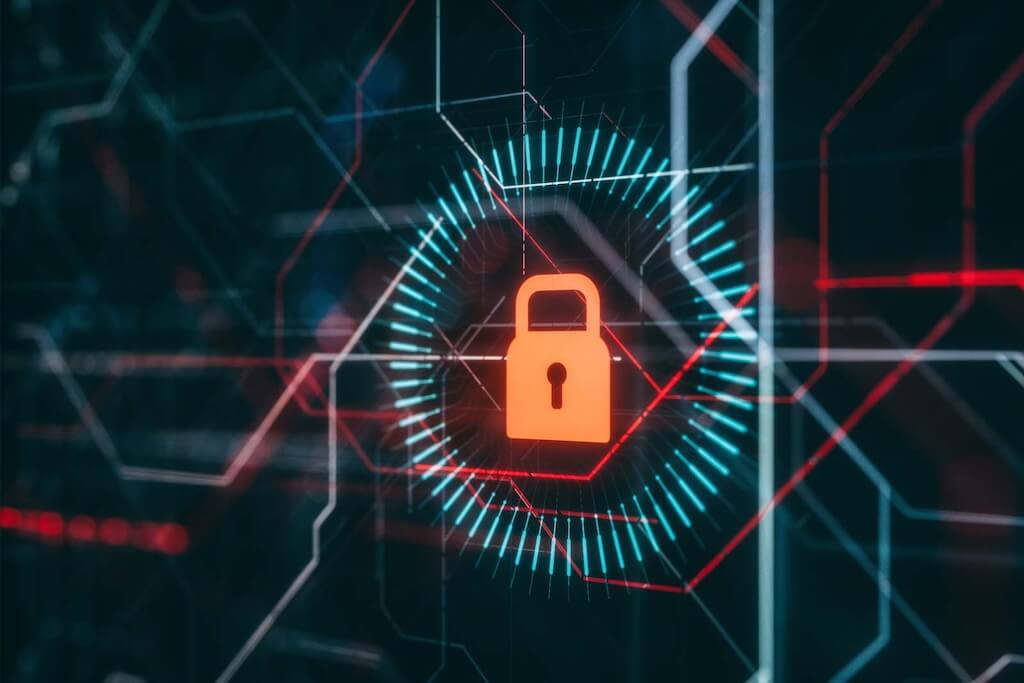
As cyber threats become more sophisticated, securing your web applications requires more than just basic protection. Advanced techniques such as Content Security Policy (CSP) and Subresource Integrity (SRI) are essential tools in the modern web developer’s arsenal. These techniques, combined with automated deployment through a CI/CD pipeline, can significantly reduce the risk of attacks such as Cross-Site Scripting (XSS) and supply chain compromises.
In this guide, we’ll explore advanced security headers, dive into the intricacies of CSP and SRI, and show you how to automate their implementation within your CI/CD pipeline. This approach will ensure that your security measures are consistently applied and updated across all environments, giving you peace of mind in an increasingly hostile digital landscape.
Advanced Security Headers
Beyond the basic security headers, there are several advanced headers that can provide additional layers of protection for your web application.
1. Content Security Policy (CSP) with Nonce-Based Script Management
CSP is a powerful tool for preventing XSS attacks by specifying the sources from which a browser can load resources. However, implementing CSP with nonces takes it a step further by dynamically generating a unique value for each request, which must match the value included in the script or style tag.
server {
listen 80;
server_name example.com;
set $nonce "123456"; # Replace with a dynamic nonce generator
add_header Content-Security-Policy "script-src 'self' 'nonce-$nonce'; object-src 'none'; base-uri 'self';";
location / {
try_files $uri $uri/ =404;
}
}
2. Subresource Integrity (SRI)
SRI ensures that the external resources you load (like JavaScript and CSS) have not been tampered with. It works by requiring a hash in the script or link tag that browsers check before executing the resource.
<link rel="stylesheet" href="https://example.com/style.css" integrity="sha384-oqVuAfXRKap7fdgcCY5uykM6+R9Gh7BfFSj3BAvlz1T1n6C29N9v93DYzA3iNWkd" crossorigin="anonymous">
<script src="https://example.com/script.js" integrity="sha384-OgVRvuATP1z7JjHLkuOUh9+J9JuFI7c2efO6GO9yM5nCrIV9aaK9z49JjQ5A4Mew" crossorigin="anonymous"></script>
3. Referrer-Policy
This header controls the amount of referrer information that is included with requests. A strict policy can prevent leaking sensitive information to third parties.
add_header Referrer-Policy "strict-origin";
Automating Security in CI/CD Pipelines
Integrating these advanced security practices into your CI/CD pipeline ensures that they are applied consistently across all deployments, reducing the risk of human error.
Step 1: Automate CSP and SRI Hash Generation
To automate the generation of CSP nonces and SRI hashes, you can use a build tool like Webpack or Gulp. Here’s an example using Webpack:
const SriPlugin = require('webpack-subresource-integrity');
const HtmlWebpackPlugin = require('html-webpack-plugin');
const { v4: uuidv4 } = require('uuid');
module.exports = {
entry: './src/index.js',
output: {
path: __dirname + '/dist',
filename: 'bundle.js',
crossOriginLoading: 'anonymous',
},
plugins: [
new HtmlWebpackPlugin({
template: './src/index.html',
nonce: uuidv4(), // Generate a unique nonce
}),
new SriPlugin({
hashFuncNames: ['sha384'],
enabled: true,
}),
],
};
Step 2: Implement Security Headers in Your CI/CD Pipeline
With tools like Jenkins, GitHub Actions, or GitLab CI, you can automate the process of applying security headers. Here’s a sample GitHub Actions workflow:
name: Deploy Application
on:
push:
branches:
- main
jobs:
deploy:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- name: Install dependencies
run: npm install
- name: Build project
run: npm run build
- name: Deploy to server
run: |
scp -r dist/* user@example.com:/var/www/example
ssh user@example.com 'sudo systemctl restart nginx'
Step 3: Automated Testing and Validation
It’s critical to test your security headers as part of your CI/CD pipeline. Integrate tools like Mozilla Observatory or Security Headers into your pipeline to automatically scan your application after each deployment.
- name: Security Headers Test
run: |
curl -s https://securityheaders.com/?q=https://example.com | grep "Grade: A"
if [ $? -ne 0 ]; then
echo "Security headers test failed"
exit 1
fi
Conclusion
Advanced web security practices like CSP, SRI, and robust security headers are essential for protecting modern web applications against sophisticated threats. By automating these practices within your CI/CD pipeline, you ensure that your security measures are consistently applied, reducing the risk of vulnerabilities slipping through the cracks.
As cyber threats continue to evolve, staying ahead of the curve with advanced security techniques is not just an option—it’s a necessity. By implementing these strategies, you’ll be well-equipped to safeguard your web applications and maintain the trust of your users.